Android - JSON Parser Tutorial
JSON stands for JavaScript Object Notation.It is an independent data exchange format and is the best alternative for XML. This chapter explains how to parse the JSON file and extract necessary information from it.
Android provides four different classes to manipulate JSON data. These classes are JSONArray,JSONObject,JSONStringer and JSONTokenizer.
The first step is to identify the fields in the JSON data in which you are interested in. For example. In the JSON given below we interested in getting temperature only.
{ "sys": { "country":"GB", "sunrise":1381107633, "sunset":1381149604 }, "weather":[ { "id":711, "main":"Smoke", "description":"smoke", "icon":"50n" } ], "main": { "temp":304.15, "pressure":1009, } }
JSON - Elements
An JSON file consist of many components. Here is the table defining the components of an JSON file and their description −
Sr.No | Component & description |
---|---|
1 | Array([)
In a JSON file , square bracket ([) represents a JSON array
|
2 | Objects({)
In a JSON file, curly bracket ({) represents a JSON object
|
3 | Key
A JSON object contains a key that is just a string. Pairs of key/value make up a JSON object
|
4 | Value
Each key has a value that could be string , integer or double e.t.c
|
JSON - Parsing
For parsing a JSON object, we will create an object of class JSONObject and specify a string containing JSON data to it. Its syntax is:
String in; JSONObject reader = new JSONObject(in);
The last step is to parse the JSON. An JSON file consist of different object with different key/value pair e.t.c. So JSONObject has a separate function for parsing each of the component of JSON file. Its syntax is given below:
JSONObject sys = reader.getJSONObject("sys"); country = sys.getString("country"); JSONObject main = reader.getJSONObject("main"); temperature = main.getString("temp");
The method getJSONObject returns the JSON object. The method getStringreturns the string value of the specified key.
Apart from the these methods , there are other methods provided by this class for better parsing JSON files. These methods are listed below −
Sr.No | Method & description |
---|---|
1 | get(String name)
This method just Returns the value but in the form of Object type
|
2 | getBoolean(String name)
This method returns the boolean value specified by the key
|
3 | getDouble(String name)
This method returns the double value specified by the key
|
4 | getInt(String name)
This method returns the integer value specified by the key
|
5 | getLong(String name)
This method returns the long value specified by the key
|
6 | length()
This method returns the number of name/value mappings in this object..
|
7 | names()
This method returns an array containing the string names in this object.
|
Example
To experiment with this example , you can run this on an actual device or in an emulator.
Steps | Description |
---|---|
1 | You will use Android studio to create an Android application. While creating this project, make sure you Target SDK and Compile With at the latest version of Android SDK to use higher levels of APIs. |
2 | Modify src/MainActivity.java file to add necessary code. |
3 | Modify the res/layout/activity_main to add respective XML components |
4 | Modify the res/values/string.xml to add necessary string components |
5 | Run the application and choose a running android device and install the application on it and verify the results |
Following is the content of the modified main activity filesrc/MainActivity.java.
package com.tutorialspoint.json; import android.app.Activity; import android.os.StrictMode; import android.support.v7.app.ActionBarActivity; import android.os.Bundle; import android.util.Log; import android.view.Menu; import android.view.MenuItem; import android.widget.Button; import android.widget.TextView; import org.apache.http.HttpEntity; import org.apache.http.HttpResponse; import org.apache.http.StatusLine; import org.apache.http.client.ClientProtocolException; import org.apache.http.client.HttpClient; import org.apache.http.client.methods.HttpGet; import org.apache.http.impl.client.DefaultHttpClient; import org.json.JSONArray; import org.json.JSONException; import org.json.JSONObject; import java.io.BufferedReader; import java.io.IOException; import java.io.InputStream; import java.io.InputStreamReader; public class MainActivity extends Activity { public void onCreate(Bundle savedInstanceState) { super.onCreate(savedInstanceState); setContentView(R.layout.activity_main); TextView output = (TextView) findViewById(R.id.textView1); String strJson=" { \"Employee\" :[ { \"id\":\"01\", \"name\":\"Gopal Varma\", \"salary\":\"500000\" }, { \"id\":\"02\", \"name\":\"Sairamkrishna\", \"salary\":\"500000\" }, { \"id\":\"03\", \"name\":\"Sathish kallakuri\", \"salary\":\"600000\" } ] }"; String data = ""; try { JSONObject jsonRootObject = new JSONObject(strJson); //Get the instance of JSONArray that contains JSONObjects JSONArray jsonArray = jsonRootObject.optJSONArray("Employee"); //Iterate the jsonArray and print the info of JSONObjects for(int i=0; i < jsonArray.length(); i++){ JSONObject jsonObject = jsonArray.getJSONObject(i); int id = Integer.parseInt(jsonObject.optString("id").toString()); String name = jsonObject.optString("name").toString(); float salary = Float.parseFloat(jsonObject.optString("salary").toString()); data += "Node"+i+" : \n id= "+ id +" \n Name= "+ name +" \n Salary= "+ salary +" \n "; } output.setText(data); } catch (JSONException e) {e.printStackTrace();} } }
Following is the modified content of the xml res/layout/activity_main.xml.
<RelativeLayout xmlns:android="http://schemas.android.com/apk/res/android" xmlns:tools="http://schemas.android.com/tools" android:layout_width="match_parent" android:layout_height="match_parent" android:paddingLeft="@dimen/activity_horizontal_margin" android:paddingRight="@dimen/activity_horizontal_margin" android:paddingTop="@dimen/activity_vertical_margin" android:paddingBottom="@dimen/activity_vertical_margin" tools:context=".MainActivity"> <TextView android:layout_width="wrap_content" android:layout_height="wrap_content" android:text="JSON example" android:id="@+id/textView" android:layout_alignParentTop="true" android:layout_centerHorizontal="true" android:textSize="30dp" /> <TextView android:layout_width="wrap_content" android:layout_height="wrap_content" android:text="Tutorials Point" android:id="@+id/textView2" android:layout_below="@+id/textView" android:layout_centerHorizontal="true" android:textSize="35dp" android:textColor="#ff16ff01" /> <TextView android:layout_width="wrap_content" android:layout_height="wrap_content" android:text="New Text" android:id="@+id/textView1" android:layout_below="@+id/textView2" android:layout_alignParentLeft="true" android:layout_alignParentStart="true" android:layout_alignParentRight="true" android:layout_alignParentEnd="true" /> </RelativeLayout>
Following is the content of AndroidManifest.xml file.
<?xml version="1.0" encoding="utf-8"?> <manifest xmlns:android="http://schemas.android.com/apk/res/android" package="com.example.sairamkrishna.myapplication" > <uses-permission android:name="android.permission.INTERNET"/> <application android:allowBackup="true" android:icon="@mipmap/ic_launcher" android:label="@string/app_name" android:theme="@style/AppTheme" > <activity android:name=".MainActivity" android:label="@string/app_name" > <intent-filter> <action android:name="android.intent.action.MAIN" /> <category android:name="android.intent.category.LAUNCHER" /> </intent-filter> </activity> </application> </manifest>
Let's try to run our application we just modified. I assume you had created yourAVD while doing environment setup. To run the app from Android studio, open one of your project's activity files and click Run
icon from the toolbar. Android studio installs the app on your AVD and starts it and if everything is fine with your setup and application, it will display following Emulator window:

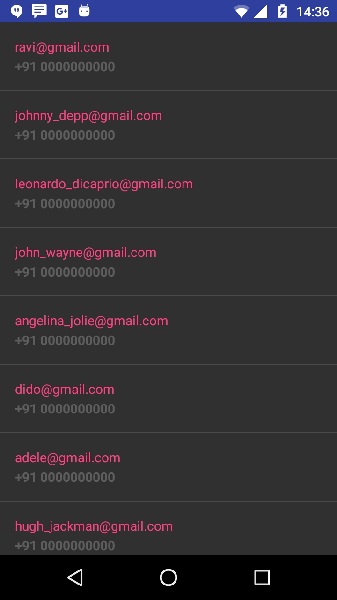
Above Example showing the data from string json,The data has contained employer details as well as salary information